Class 26: More Clustering ModelsΒΆ
# %load http://drsmb.co/310
import matplotlib.pyplot as plt
import numpy as np
import seaborn as sns
from sklearn import datasets
from sklearn import cluster
from sklearn import metrics
iris_X, iris_y = datasets.load_iris(return_X_y = True)
msc = cluster.MeanShift()
msc.fit(iris_X)
MeanShift()
plt.scatter(iris_X[:,0],iris_X[:,1], c= msc.labels_)
<matplotlib.collections.PathCollection at 0x7f4f1d1d2110>
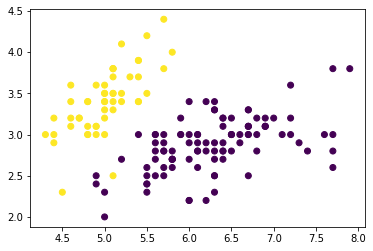
sns.pairplot(iris_X, hue= msc.labels_)
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
<ipython-input-6-7e1512556191> in <module>
----> 1 sns.pairplot(iris_X, hue= msc.labels_)
/opt/hostedtoolcache/Python/3.7.10/x64/lib/python3.7/site-packages/seaborn/_decorators.py in inner_f(*args, **kwargs)
44 )
45 kwargs.update({k: arg for k, arg in zip(sig.parameters, args)})
---> 46 return f(**kwargs)
47 return inner_f
48
/opt/hostedtoolcache/Python/3.7.10/x64/lib/python3.7/site-packages/seaborn/axisgrid.py in pairplot(data, hue, hue_order, palette, vars, x_vars, y_vars, kind, diag_kind, markers, height, aspect, corner, dropna, plot_kws, diag_kws, grid_kws, size)
1972 raise TypeError(
1973 "'data' must be pandas DataFrame object, not: {typefound}".format(
-> 1974 typefound=type(data)))
1975
1976 plot_kws = {} if plot_kws is None else plot_kws.copy()
TypeError: 'data' must be pandas DataFrame object, not: <class 'numpy.ndarray'>
spc = cluster.SpectralClustering(n_clusters=3)
spc.fit(iris_X)
SpectralClustering(n_clusters=3)
plt.scatter(iris_X[:,0],iris_X[:,1], c=spc.labels_)
<matplotlib.collections.PathCollection at 0x7f4f11874990>
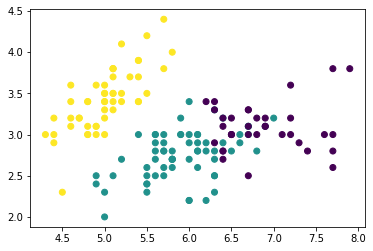
km3 = cluster.KMeans(n_clusters=3)
km3.fit(iris_X)
plt.scatter(iris_X[:,0],iris_X[:,1], c=km3.labels_)
<matplotlib.collections.PathCollection at 0x7f4f11807b50>
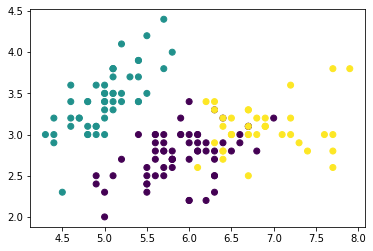
from sklearn.neighbors import NearestNeighbors
nbrs = NearestNeighbors()
nbrs.fit(iris_X)
NearestNeighbors()
dists, indx = nbrs.kneighbors(iris_X)
dists
array([[0. , 0.1 , 0.14142136, 0.14142136, 0.14142136],
[0. , 0.14142136, 0.14142136, 0.14142136, 0.17320508],
[0. , 0.14142136, 0.24494897, 0.26457513, 0.26457513],
[0. , 0.14142136, 0.17320508, 0.2236068 , 0.24494897],
[0. , 0.14142136, 0.14142136, 0.17320508, 0.17320508],
[0. , 0.33166248, 0.34641016, 0.36055513, 0.37416574],
[0. , 0.2236068 , 0.26457513, 0.3 , 0.31622777],
[0. , 0.1 , 0.14142136, 0.17320508, 0.2 ],
[0. , 0.14142136, 0.3 , 0.31622777, 0.34641016],
[0. , 0.1 , 0.17320508, 0.17320508, 0.17320508],
[0. , 0.1 , 0.28284271, 0.3 , 0.33166248],
[0. , 0.2236068 , 0.2236068 , 0.28284271, 0.3 ],
[0. , 0.14142136, 0.17320508, 0.2 , 0.2 ],
[0. , 0.24494897, 0.31622777, 0.34641016, 0.47958315],
[0. , 0.41231056, 0.46904158, 0.54772256, 0.55677644],
[0. , 0.36055513, 0.54772256, 0.6164414 , 0.6164414 ],
[0. , 0.34641016, 0.36055513, 0.38729833, 0.38729833],
[0. , 0.1 , 0.14142136, 0.17320508, 0.17320508],
[0. , 0.33166248, 0.38729833, 0.46904158, 0.50990195],
[0. , 0.14142136, 0.14142136, 0.24494897, 0.26457513],
[0. , 0.28284271, 0.3 , 0.36055513, 0.36055513],
[0. , 0.14142136, 0.24494897, 0.24494897, 0.26457513],
[0. , 0.45825757, 0.50990195, 0.50990195, 0.51961524],
[0. , 0.2 , 0.26457513, 0.37416574, 0.38729833],
[0. , 0.3 , 0.37416574, 0.41231056, 0.42426407],
[0. , 0.17320508, 0.2 , 0.2236068 , 0.2236068 ],
[0. , 0.2 , 0.2236068 , 0.2236068 , 0.24494897],
[0. , 0.14142136, 0.14142136, 0.14142136, 0.17320508],
[0. , 0.14142136, 0.14142136, 0.14142136, 0.17320508],
[0. , 0.14142136, 0.17320508, 0.2236068 , 0.2236068 ],
[0. , 0.14142136, 0.14142136, 0.17320508, 0.2236068 ],
[0. , 0.28284271, 0.3 , 0.3 , 0.31622777],
[0. , 0.34641016, 0.34641016, 0.37416574, 0.42426407],
[0. , 0.34641016, 0.36055513, 0.38729833, 0.41231056],
[0. , 0.1 , 0.14142136, 0.14142136, 0.17320508],
[0. , 0.2236068 , 0.3 , 0.31622777, 0.33166248],
[0. , 0.3 , 0.31622777, 0.33166248, 0.34641016],
[0. , 0.14142136, 0.24494897, 0.26457513, 0.26457513],
[0. , 0.14142136, 0.2 , 0.24494897, 0.3 ],
[0. , 0.1 , 0.14142136, 0.14142136, 0.14142136],
[0. , 0.14142136, 0.17320508, 0.17320508, 0.24494897],
[0. , 0.6244998 , 0.71414284, 0.76811457, 0.78102497],
[0. , 0.2 , 0.2236068 , 0.3 , 0.3 ],
[0. , 0.2236068 , 0.26457513, 0.31622777, 0.37416574],
[0. , 0.36055513, 0.37416574, 0.41231056, 0.41231056],
[0. , 0.14142136, 0.2 , 0.2 , 0.24494897],
[0. , 0.14142136, 0.24494897, 0.24494897, 0.3 ],
[0. , 0.14142136, 0.14142136, 0.2236068 , 0.2236068 ],
[0. , 0.1 , 0.2236068 , 0.24494897, 0.24494897],
[0. , 0.14142136, 0.17320508, 0.2236068 , 0.2236068 ],
[0. , 0.26457513, 0.33166248, 0.43588989, 0.45825757],
[0. , 0.26457513, 0.31622777, 0.34641016, 0.37416574],
[0. , 0.26457513, 0.28284271, 0.31622777, 0.34641016],
[0. , 0.2 , 0.3 , 0.31622777, 0.43588989],
[0. , 0.24494897, 0.31622777, 0.37416574, 0.37416574],
[0. , 0.3 , 0.31622777, 0.31622777, 0.33166248],
[0. , 0.26457513, 0.37416574, 0.42426407, 0.45825757],
[0. , 0.14142136, 0.38729833, 0.45825757, 0.72111026],
[0. , 0.24494897, 0.24494897, 0.31622777, 0.31622777],
[0. , 0.38729833, 0.50990195, 0.51961524, 0.52915026],
[0. , 0.36055513, 0.45825757, 0.67082039, 0.71414284],
[0. , 0.3 , 0.33166248, 0.36055513, 0.36055513],
[0. , 0.48989795, 0.51961524, 0.54772256, 0.58309519],
[0. , 0.14142136, 0.2236068 , 0.24494897, 0.42426407],
[0. , 0.42426407, 0.4472136 , 0.50990195, 0.51961524],
[0. , 0.14142136, 0.31622777, 0.31622777, 0.34641016],
[0. , 0.2 , 0.3 , 0.38729833, 0.41231056],
[0. , 0.24494897, 0.28284271, 0.33166248, 0.36055513],
[0. , 0.26457513, 0.50990195, 0.53851648, 0.678233 ],
[0. , 0.17320508, 0.24494897, 0.26457513, 0.26457513],
[0. , 0.2236068 , 0.3 , 0.36055513, 0.42426407],
[0. , 0.33166248, 0.34641016, 0.37416574, 0.4 ],
[0. , 0.36055513, 0.36055513, 0.41231056, 0.42426407],
[0. , 0.2236068 , 0.3 , 0.38729833, 0.43588989],
[0. , 0.2 , 0.26457513, 0.36055513, 0.38729833],
[0. , 0.14142136, 0.24494897, 0.26457513, 0.31622777],
[0. , 0.31622777, 0.34641016, 0.34641016, 0.37416574],
[0. , 0.31622777, 0.37416574, 0.41231056, 0.42426407],
[0. , 0.2 , 0.24494897, 0.33166248, 0.34641016],
[0. , 0.34641016, 0.42426407, 0.43588989, 0.4472136 ],
[0. , 0.14142136, 0.17320508, 0.3 , 0.3 ],
[0. , 0.14142136, 0.26457513, 0.34641016, 0.43588989],
[0. , 0.14142136, 0.26457513, 0.28284271, 0.3 ],
[0. , 0.33166248, 0.36055513, 0.36055513, 0.37416574],
[0. , 0.2 , 0.41231056, 0.47958315, 0.48989795],
[0. , 0.37416574, 0.42426407, 0.45825757, 0.46904158],
[0. , 0.28284271, 0.31622777, 0.31622777, 0.33166248],
[0. , 0.26457513, 0.57445626, 0.59160798, 0.60827625],
[0. , 0.17320508, 0.17320508, 0.2236068 , 0.31622777],
[0. , 0.2 , 0.24494897, 0.3 , 0.3 ],
[0. , 0.26457513, 0.31622777, 0.42426407, 0.42426407],
[0. , 0.14142136, 0.2 , 0.3 , 0.34641016],
[0. , 0.14142136, 0.24494897, 0.26457513, 0.26457513],
[0. , 0.14142136, 0.36055513, 0.38729833, 0.64807407],
[0. , 0.17320508, 0.2236068 , 0.26457513, 0.3 ],
[0. , 0.14142136, 0.17320508, 0.24494897, 0.33166248],
[0. , 0.14142136, 0.14142136, 0.17320508, 0.2236068 ],
[0. , 0.2 , 0.33166248, 0.34641016, 0.34641016],
[0. , 0.38729833, 0.38729833, 0.72111026, 0.79372539],
[0. , 0.14142136, 0.17320508, 0.2236068 , 0.24494897],
[0. , 0.42426407, 0.5 , 0.50990195, 0.55677644],
[0. , 0. , 0.26457513, 0.31622777, 0.33166248],
[0. , 0.38729833, 0.4 , 0.41231056, 0.45825757],
[0. , 0.24494897, 0.24494897, 0.33166248, 0.38729833],
[0. , 0.3 , 0.31622777, 0.36055513, 0.38729833],
[0. , 0.26457513, 0.52915026, 0.54772256, 0.54772256],
[0. , 0.73484692, 0.76157731, 0.79372539, 0.87749644],
[0. , 0.26457513, 0.43588989, 0.52915026, 0.54772256],
[0. , 0.55677644, 0.6 , 0.6164414 , 0.6164414 ],
[0. , 0.63245553, 0.67082039, 0.70710678, 0.75498344],
[0. , 0.2236068 , 0.37416574, 0.42426407, 0.42426407],
[0. , 0.34641016, 0.37416574, 0.37416574, 0.38729833],
[0. , 0.17320508, 0.34641016, 0.36055513, 0.37416574],
[0. , 0.26457513, 0.26457513, 0.33166248, 0.51961524],
[0. , 0.48989795, 0.50990195, 0.50990195, 0.51961524],
[0. , 0.3 , 0.37416574, 0.37416574, 0.38729833],
[0. , 0.14142136, 0.24494897, 0.36055513, 0.38729833],
[0. , 0.41231056, 0.81853528, 0.86023253, 1.00498756],
[0. , 0.41231056, 0.54772256, 0.89442719, 0.92736185],
[0. , 0.43588989, 0.51961524, 0.53851648, 0.58309519],
[0. , 0.2236068 , 0.26457513, 0.3 , 0.3 ],
[0. , 0.31622777, 0.31622777, 0.33166248, 0.45825757],
[0. , 0.26457513, 0.41231056, 0.60827625, 0.678233 ],
[0. , 0.17320508, 0.24494897, 0.36055513, 0.36055513],
[0. , 0.3 , 0.31622777, 0.37416574, 0.37416574],
[0. , 0.34641016, 0.38729833, 0.43588989, 0.46904158],
[0. , 0.17320508, 0.24494897, 0.28284271, 0.38729833],
[0. , 0.14142136, 0.24494897, 0.28284271, 0.3 ],
[0. , 0.1 , 0.31622777, 0.33166248, 0.37416574],
[0. , 0.34641016, 0.50990195, 0.51961524, 0.55677644],
[0. , 0.26457513, 0.45825757, 0.46904158, 0.50990195],
[0. , 0.41231056, 0.88317609, 0.92736185, 0.93273791],
[0. , 0.1 , 0.3 , 0.42426407, 0.43588989],
[0. , 0.33166248, 0.36055513, 0.37416574, 0.43588989],
[0. , 0.53851648, 0.55677644, 0.58309519, 0.66332496],
[0. , 0.53851648, 0.54772256, 0.66332496, 0.678233 ],
[0. , 0.24494897, 0.38729833, 0.42426407, 0.43588989],
[0. , 0.14142136, 0.24494897, 0.38729833, 0.43588989],
[0. , 0.14142136, 0.2236068 , 0.28284271, 0.31622777],
[0. , 0.17320508, 0.36055513, 0.36055513, 0.37416574],
[0. , 0.24494897, 0.26457513, 0.34641016, 0.34641016],
[0. , 0.24494897, 0.36055513, 0.46904158, 0.50990195],
[0. , 0. , 0.26457513, 0.31622777, 0.33166248],
[0. , 0.2236068 , 0.31622777, 0.31622777, 0.34641016],
[0. , 0.24494897, 0.3 , 0.31622777, 0.4 ],
[0. , 0.24494897, 0.36055513, 0.36055513, 0.37416574],
[0. , 0.24494897, 0.37416574, 0.38729833, 0.41231056],
[0. , 0.2236068 , 0.34641016, 0.36055513, 0.36055513],
[0. , 0.24494897, 0.3 , 0.55677644, 0.6164414 ],
[0. , 0.28284271, 0.31622777, 0.33166248, 0.33166248]])
indx
array([[ 0, 17, 4, 39, 27],
[ 1, 34, 45, 12, 9],
[ 2, 47, 3, 12, 6],
[ 3, 47, 29, 30, 2],
[ 4, 37, 0, 17, 40],
[ 5, 18, 10, 48, 44],
[ 6, 47, 2, 11, 42],
[ 7, 39, 49, 0, 17],
[ 8, 38, 3, 42, 13],
[ 9, 34, 1, 30, 12],
[ 10, 48, 27, 36, 19],
[ 11, 29, 7, 26, 24],
[ 12, 1, 9, 45, 34],
[ 13, 38, 42, 8, 47],
[ 14, 33, 16, 15, 18],
[ 15, 33, 14, 5, 16],
[ 16, 10, 48, 33, 19],
[ 17, 0, 40, 4, 39],
[ 18, 5, 10, 48, 20],
[ 19, 21, 46, 48, 4],
[ 20, 31, 27, 28, 10],
[ 21, 19, 46, 17, 4],
[ 22, 6, 2, 37, 40],
[ 23, 26, 43, 39, 7],
[ 24, 11, 29, 26, 30],
[ 25, 34, 9, 1, 30],
[ 26, 23, 43, 7, 39],
[ 27, 28, 0, 39, 17],
[ 28, 27, 0, 39, 17],
[ 29, 30, 3, 11, 47],
[ 30, 29, 34, 9, 25],
[ 31, 20, 28, 27, 36],
[ 32, 46, 33, 19, 48],
[ 33, 32, 15, 16, 14],
[ 34, 9, 1, 30, 25],
[ 35, 49, 1, 2, 40],
[ 36, 10, 31, 28, 48],
[ 37, 4, 0, 40, 7],
[ 38, 8, 42, 13, 3],
[ 39, 7, 0, 28, 27],
[ 40, 17, 0, 4, 7],
[ 41, 8, 38, 45, 13],
[ 42, 38, 47, 3, 2],
[ 43, 26, 23, 21, 17],
[ 44, 46, 5, 21, 19],
[ 45, 1, 12, 34, 30],
[ 46, 19, 21, 48, 4],
[ 47, 3, 2, 42, 6],
[ 48, 10, 27, 19, 46],
[ 49, 7, 39, 35, 0],
[ 50, 52, 86, 65, 76],
[ 51, 56, 75, 65, 86],
[ 52, 50, 86, 77, 76],
[ 53, 89, 80, 69, 81],
[ 54, 58, 75, 76, 86],
[ 55, 66, 90, 96, 94],
[ 56, 51, 85, 91, 127],
[ 57, 93, 98, 60, 81],
[ 58, 75, 54, 65, 76],
[ 59, 89, 94, 53, 80],
[ 60, 93, 57, 81, 80],
[ 61, 96, 78, 95, 99],
[ 62, 92, 69, 67, 80],
[ 63, 91, 73, 78, 97],
[ 64, 82, 79, 88, 99],
[ 65, 75, 58, 86, 51],
[ 66, 84, 55, 96, 78],
[ 67, 92, 82, 99, 69],
[ 68, 87, 72, 119, 54],
[ 69, 80, 89, 81, 92],
[ 70, 138, 127, 149, 85],
[ 71, 97, 82, 92, 61],
[ 72, 133, 123, 146, 83],
[ 73, 63, 91, 78, 97],
[ 74, 97, 75, 58, 54],
[ 75, 65, 58, 74, 54],
[ 76, 58, 86, 52, 54],
[ 77, 52, 86, 147, 110],
[ 78, 91, 63, 61, 97],
[ 79, 81, 80, 69, 64],
[ 80, 81, 69, 89, 53],
[ 81, 80, 69, 79, 89],
[ 82, 92, 99, 67, 69],
[ 83, 133, 101, 142, 149],
[ 84, 66, 55, 96, 88],
[ 85, 56, 70, 51, 91],
[ 86, 52, 65, 58, 50],
[ 87, 68, 72, 62, 54],
[ 88, 96, 95, 99, 94],
[ 89, 53, 69, 80, 94],
[ 90, 94, 55, 96, 89],
[ 91, 63, 78, 73, 97],
[ 92, 82, 67, 99, 69],
[ 93, 57, 60, 98, 81],
[ 94, 99, 96, 90, 89],
[ 95, 96, 88, 99, 94],
[ 96, 95, 99, 88, 94],
[ 97, 74, 71, 91, 78],
[ 98, 57, 93, 60, 79],
[ 99, 96, 94, 88, 95],
[100, 136, 144, 104, 143],
[101, 142, 113, 121, 149],
[102, 125, 120, 143, 130],
[103, 116, 137, 128, 111],
[104, 132, 128, 140, 124],
[105, 122, 107, 135, 118],
[106, 84, 59, 90, 89],
[107, 130, 125, 105, 102],
[108, 128, 103, 132, 116],
[109, 143, 120, 144, 102],
[110, 147, 115, 77, 145],
[111, 147, 128, 146, 103],
[112, 139, 140, 120, 145],
[113, 101, 142, 121, 114],
[114, 121, 101, 142, 113],
[115, 148, 145, 110, 147],
[116, 137, 103, 147, 111],
[117, 131, 105, 109, 135],
[118, 122, 105, 135, 107],
[119, 72, 83, 68, 146],
[120, 143, 140, 124, 144],
[121, 101, 142, 113, 149],
[122, 105, 118, 107, 130],
[123, 126, 146, 127, 72],
[124, 120, 143, 140, 112],
[125, 129, 102, 107, 130],
[126, 123, 127, 138, 146],
[127, 138, 126, 149, 70],
[128, 132, 104, 103, 111],
[129, 125, 130, 102, 107],
[130, 107, 102, 125, 129],
[131, 117, 105, 135, 109],
[132, 128, 104, 103, 111],
[133, 83, 72, 123, 126],
[134, 103, 83, 133, 111],
[135, 130, 105, 102, 107],
[136, 148, 115, 100, 144],
[137, 116, 103, 147, 128],
[138, 127, 70, 126, 149],
[139, 112, 145, 141, 120],
[140, 144, 120, 112, 143],
[141, 145, 139, 112, 110],
[101, 142, 113, 121, 149],
[143, 120, 124, 144, 140],
[144, 140, 120, 143, 124],
[145, 141, 147, 139, 112],
[146, 123, 111, 126, 72],
[147, 110, 111, 116, 145],
[148, 136, 115, 110, 147],
[149, 127, 138, 101, 142]])